Introduction
HTTP Basic Authentication is an authentication method that’s built into the HTTP spec. It can be a very easy means of implementing authentication on a web application, and is a common way to secure non-production environments like QA and Staging environments without having to add an application-level auth mechanism.
Browsers have built-in support for supporting HTTP Basic Authentication. When a browser request receives an
HTTP 401 Unauthorized
response code, together with a WWW-Authenticate: Basic realm="<realm>"
response header field
(where <realm>
can be any user-defined string), it will automatically prompt the user to enter a username and
password. When the user enters their credentials, the request will be re-issued with the credentials passed via an
Authorization
request header and, if the credentials are correct, the user will be authorized to access the protected
page.
How HTTP Basic Authentication works
There are two methods of authenticating via Basic Auth:
- Passing credentials in the URL.
- Using
Authorization
request header.
Method 1: Passing credentials via URL
Authentication credentials can be passed directly in the URL, allowing you to bypass the browser prompt. To pass authentication credentials in the URL, you must use the following format:
|
|
The username and password are separated by a colon (:), then an @ is placed before the URL to be requested.
If the username and password contains : and @ in their values, you should first urlencode the values.
Example in Javascript:
|
|
Method 2: Using the Authorization HTTP request header
In situations where you’re making an API call directly, such as in an integration test or when using a utility like
curl
, credentials can be populated directly in the Authorization
HTTP request header via the following steps:
- Create a string combining the username and password separated by a colon (:) i.e username:password.
- This string should be base64 encoded
- The authorization header should be constructed as follows:
Authorization: Basic [encoded string]
Making it work in Selenium
Let’s create a Selenium script that interacts with a site that’s secured via HTTP Basic Authentication. This article assumes you are already fairly familiar with Selenium. For a detailed introduction into using Selenium, check the official documentation as well as our tutorial on writing end-to-end tests with Selenium.
Requirements
- Selenium
- Node (Since we will be using Selenium’s JavaScript library)
- npm
- Chrome WebDriver
Create a new NPM project using npm init
and follow through the prompt.
Your package.json
file should be looking like this
|
|
Install Selenium using npm install selenium-webdriver
Writing the test
Because Selenium does not natively provide us with a way to modify the headers of a request, we will be making our test by passing the credentials via the URL.
|
|
When the browser is launched, if our credentials is valid, we should see a success screen
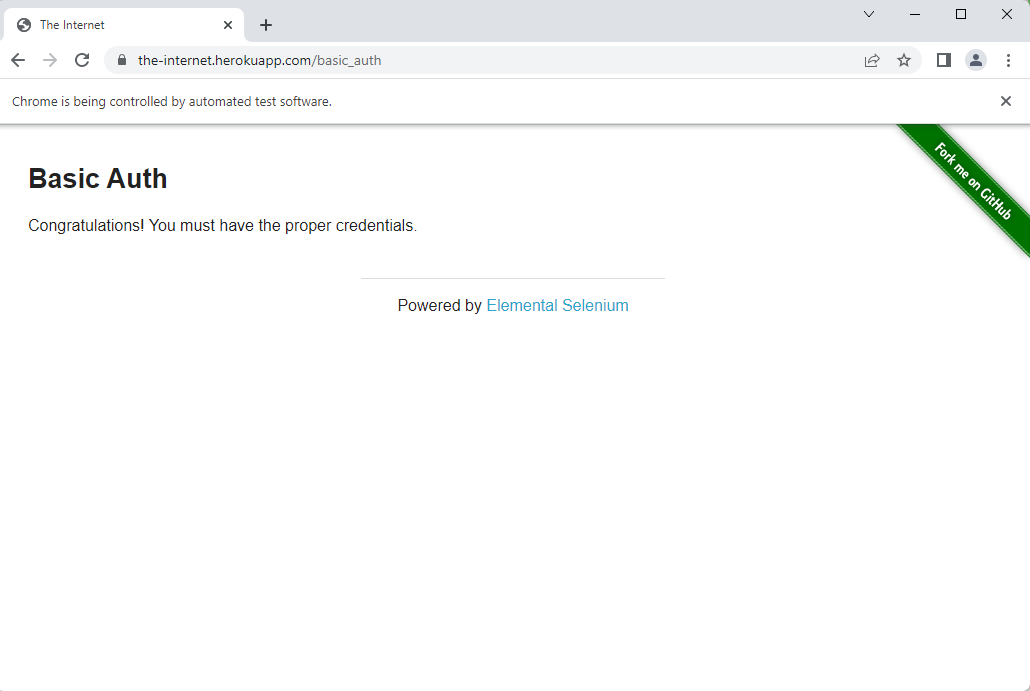
But if we supplied invalid credentials, we will be prompted for a username and password as seen below:
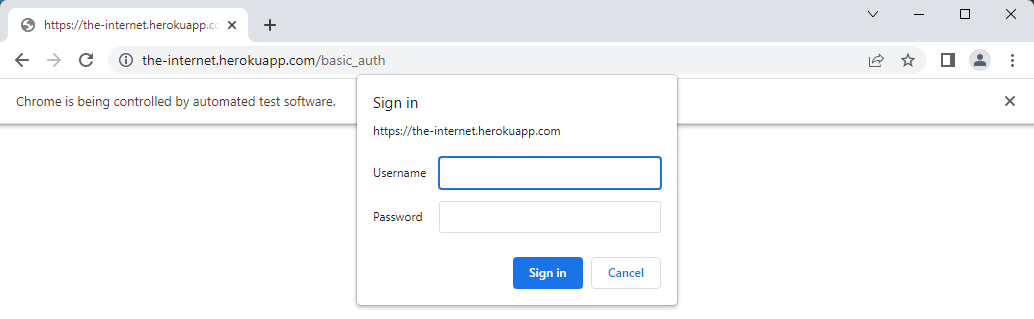
Note on Security
The Basic Authentication mechanism does not provide confidentiality on the data transmitted since the credentials are transmitted in plain text. At best, the credentials are base64 encoded which can easily be decoded. If you’re authenticating via Basic Auth, you should do so over HTTPS to ensure both the user credentials as well as the contents of the protected site are not visible to malicious third parties.