Many web applications have functionality that generates automated emails. These emails often represent critical parts of a user’s journey through the application, and any bugs affecting them could have serious negative implications.
One of most common workflows where automated emails are used are new user registration scenarios. Often after signing up for an account, you’ll be asked to confirm your email address via an automated email that sends a confirmation email or by entering a short verification code (which is sometimes called a one-time password or OTP for short).
In this article, you will learn how to create an automated test for such an email workflow using Selenium WebDriver. Specifically, we’ll create a Selenium test in Java that fills out a registration form, waits for an email to arrive at a given email address, extracts the confirmation URL from the email, and visits that URL in the browser to complete the registration process.
What are the common scenarios that include automated emails?
There are many potential end-to-end scenarios that could involve sending automated emails. Here are a few of the most common end-to-end scenarios that span both web and email:
- Forgot password: In this scenario, an email is sent to a user to allow them regain access to a website when they’ve lost their password. These emails generally involve a password recovery link.
- Passwordless authentication: The email sent to a user to allow them to access a website without entering a password. These emails contain a unique link (sometimes called a “magic link”) that automatically authenticates the user.
- New user registration: The scenario where a user is prompted to validate their email address before their new account is fully registered. The email usually contains a unique link that takes the user back to the application, but can sometimes include a short code that the user must enter to complete registration.
- Two-factor challenges: For web applications that support two-factor authentication (2FA), after entering a valid username and password, the user will be prompted to complete a “challenge” which verifies their identity. The second “factor” refers to a second medium outside of the web application itself which is used to deliver the challenge. If the second factor is email, then the user will be required to either click a link in the email, or copy a code from the email.
Why You Should Test Your Email Workflows
There are several reasons why email workflows are important for your website, and you should test them accordingly. Let’s dive into the three most important ones.
- They save you time: Email workflows are automatic and allow your users to accomplish a goal on their own, saving you from manually dealing with tedious tasks. This is true only if your email workflows are easy to follow and work as expected.
- They provide an individual and personalized approach: Email workflows can use the data you collected from your users to offer them a unique and personalized experience. If you are using personalization to customize your email workflows, it’s even more critical to be leveraging test automation. This is because the more personalized your email workflows are, the more test cases you will need to have if you want to achieve good coverage.
- They support your user: Email workflows automatically assist your users, offering them a hand when they need it. Verifying the effectiveness of the help offered to the users through testing is essential.
How To Test an Email Workflow with Selenium
Let’s now learn how to test a registration email workflow with Selenium in a step-by-step tutorial based on a complete example. Thanks to Selenium, you can retrieve the URL provided to the newly subscribed user via email to see if it matches the expected one in only a few lines of code.
1. Connecting to the Registration Page
First, you have to use Selenium to connect to the registration page of the website you want to test the email workflow. You can achieve this as follows:
|
|
This is what http://localhost/registration-page
looks like:
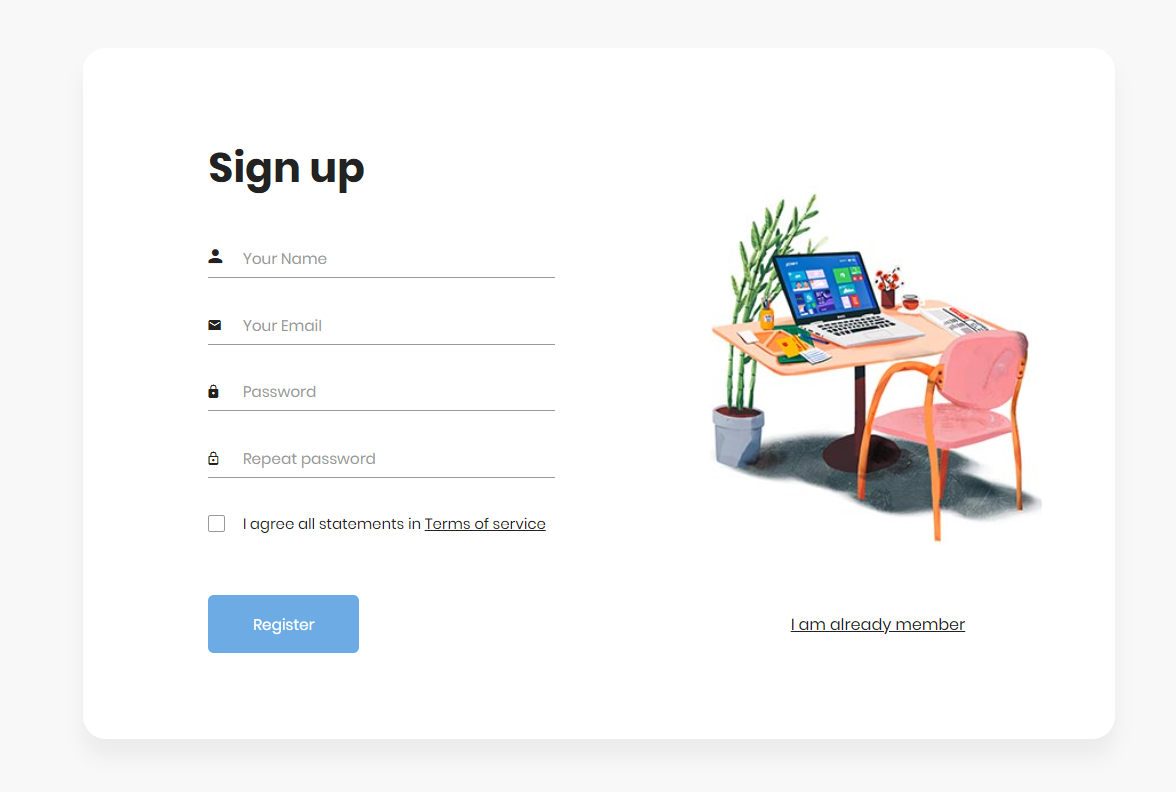
As you can see, it is a simple registration form based on a
free Bootstrap 5 template. In a real-world test, make sure to
replace http://localhost/registration-page
with the URL of your registration page.
2. Filling out the registration form
Now, it is time to fill out the registration form and submit it in Selenium to trigger the email workflow.
This is what the sample HTML form looks like:
|
|
So, the Selenium script should implement the following logic:
|
|
Keep in mind that you have to adapt this logic according to the layout of your registration page. Also, note that the
email provided to the registration form via Selenium is a 10-minute email address. You can retrieve this from the
email
input by minuteinbox.com. This is what the minuteinbox.com page looks like:
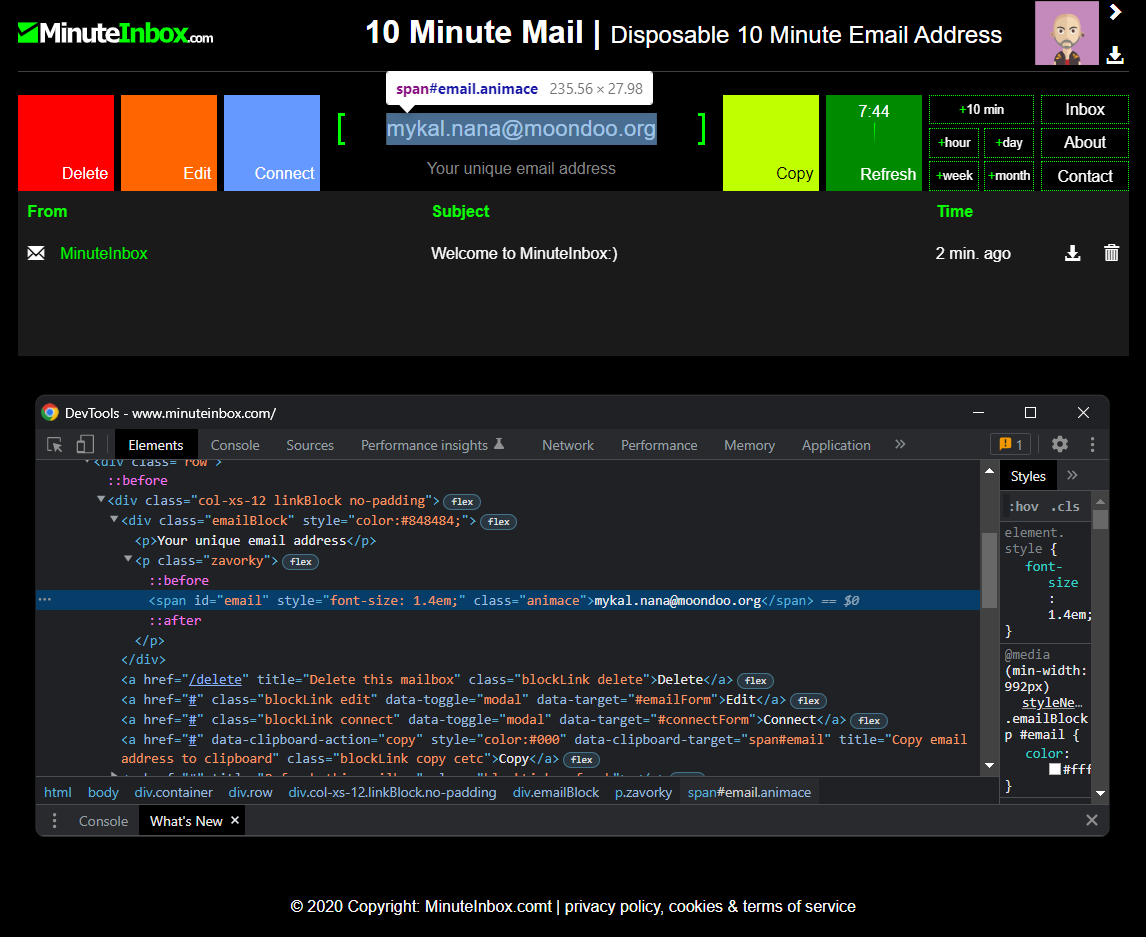
A 10-minute mail is a disposable email address that self-destructs after 10 minutes. A 10-minute email provider offers
everything you need to receive emails. Since the email containing the confirmation link will be received here, you have
to keep this window open. This is why the Selenium script initializes two different
WebDriver
instances.
Using a 10-minute email is a good way to test an email workflow. This is because most popular email providers such as Gmail have logic that detects and block automated scripts such as Selenium. On the other hand, minuteinbox.com is a simple service, does not require authentication, and is Selenium-friendly.
3. Retrieving the Confirmation Email
You now have to use Selenium to interact with the 10-minute email provider page, find the confirmation email, and open it.
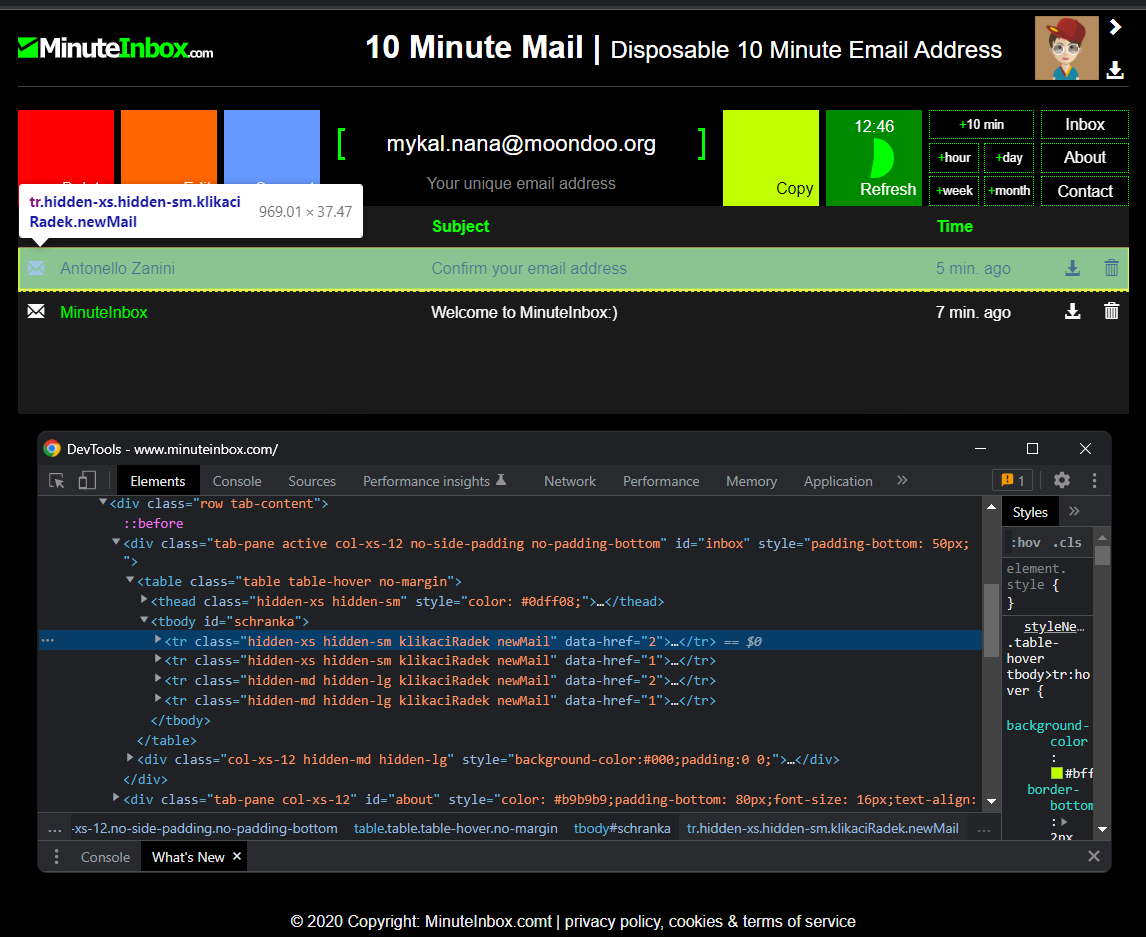
To do so, you have to click on <tr class='hidden-xs hidden-sm klikaciRadek newMail``"
data-href=``"``2>
, wait
for the iframeMail
<iframe>
element in the new page to load, and extract the text of the email from its body. You
can achieve this as follows:
|
|
The confirmation email might need minutes to arrive at your 10-minute email address. If you want to avoid a
NoSuchElementException
,
you can wait a few minutes for the email with
WebDriverWait
.
Note that we have a separate article with
tips for avoiding NoSuchElementException errors
in Selenium.
4. Extracting the URL of the Confirmation Link From the Email
This is what the confirmation email looks like:
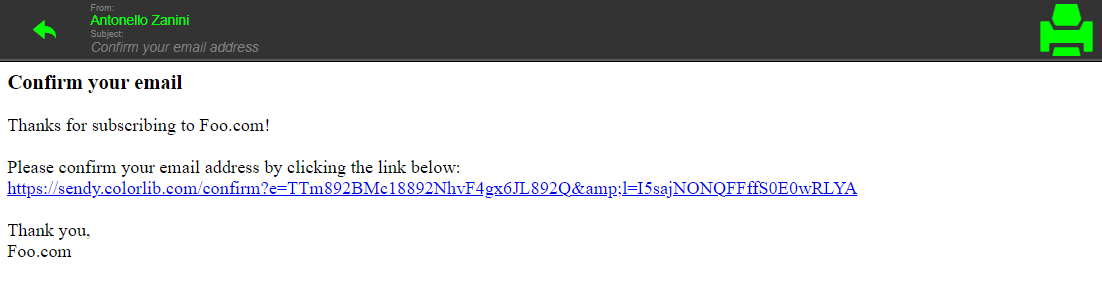
To retrieve the URL of the confirmation link, you have to extract it from the
HTML text node of the iframe’s body
previously
selected. Achieve this as follows:
|
|
The URL can be retrieved by extracting the text contained between “the link below:” and “Thank you” with the
substring(int beginIndex, int endIndex)
method.
Putting It All Together
This is what the entire Selenium script looks like:
|
|
With the confirmation email seen before, this script would return:
https://sendy.colorlib.com/confirm?e=TTm892BMc18892NhvF4gx6JL892Q&l=I5sajNONQFFffS0E0wRLYA
Et voilà! You just retrieved the URL to visit to confirm the email address of the user. For information how to achieve test coverage for SMS, check out our article on testing SMS workflows using Selenium WebDriver.
Conclusion
In this article, you learned what e-mail workflows are, why testing them is so important, and why the URLs contained in these e-mails are what matter most when it comes to testing them. Considering how common e-mail workflows are, being able to test them is critical. Here, you learned how to implement a Selenium WebDriver test that triggers the e-mail workflow after a user registers with a website. Then, you saw how to use Selenium to retrieve the URL of the confirmation email sent to the user’s inbox and test whether it matches the expected URL.
Reflect: A testing tool with built-in support for testing email workflows
Reflect is a no-code testing tool that can test virtually any action you can take on a browser. Creating a test in Reflect is easy: the tool records your actions as you use your site and automatically translates those actions into a repeatable test that you can run any time. And with its email testing feature, you can create end-to-end tests that span web and email in a matter of minutes.
Get automated test coverage for your app today — try it for free.