Many web applications now rely on your phone number for authentication or to deliver critical information. Specifically, these applications tend to send SMS messages to your personal phone number. Considering how rarely a person changes their phone number in life and how much we check our phones, SMS messages are one of the best ways to authenticate a user, get in touch with them, or communicate something urgent.
One of the most common workflows where automatic SMS is used is when a user logs in to a service. If two-factor authentication is enabled, an automated SMS containing a secure code is sent to the phone number of the user. Similarly, automated SMS workflows are used when a new user registers with an online service. Registration forms frequently ask for a personal phone number. After signing up, you will be asked to confirm your phone number via an automated SMS that contains a short verification code (often called a one-time password or OTP).
In this article, you will learn how to create an automated test for such an SMS workflow using Selenium WebDriver. Specifically, you will see how to create a Selenium test in Java that fills out a registration form involving a phone number field, waits for an SMS to arrive at the given phone number, extracts the verification code from the SMS, and uses the extracted code to complete the registration process.
What are the common scenarios that include automated SMS messages?
There are several possible end-to-end scenarios where automated SMS messages are used. Here is a list of the most common scenarios that spans both web and SMS:
- Passwordless authentication: Here, the SMS is sent to the phone number of the user to allow them to log in to a website without manually entering a password. These SMS messages generally contain a unique link (often called a “magic link”) that automatically authenticates the user after clicking on it.
- Password recovery: In this scenario, an automated SMS is sent to the phone number of the user to allow them to regain access to a website after they lost their password. Such SMS messages generally involve a password recovery link.
- New user registration: After signing up, a user is prompted to validate their phone number before their new account is activated through an automated SMS. This SMS usually contains a verification code that the user must enter to complete registration or a unique link that logs the user in.
- Two-factor challenges: when a web application supports two-factor authentication (2FA) and the user enables it, after entering a valid username and password, the user will be asked to complete a “challenge” to verify their identity. The second “factor” refers to a second medium outside the web application itself, which is used to deliver the challenge. If the second factor is SMS, then the user will be required to enter the verification code contained in the SMS or click on a link received through it.
Why you should test your SMS workflows
There are several reasons why SMS workflows are critical for your website or web application, and you should incorporate them into your overall regression testing strategy. Let’s dig into the three most important ones.
- They communicate critical information your user: SMS workflows automatically support your users by providing them with vital information directly on their phone, which is the most popular and common communication device. This is useful for automatically notifying your users when something suspicious happens. Testing is the only way to ensure that this critical information is delivered as intended.
- They save you time: SMS workflows are automated, which means that they allow your users to accomplish a goal on their own, with no external human interaction required. This prevents you from dealing with tedious tasks, but only if your SMS workflows work as expected.
- They enable a personalized approach: SMS workflows can exploit the data you collected from your users to offer them a personalized and targeted experience. If you are customizing your automated SMS messages, testing them is even more critical. The reason is that the more personalized your SMS workflows are, the more test cases you will be required to achieve good coverage.
How To Test an SMS Workflow with Selenium
Let’s now learn how to test a registration SMS workflow with Selenium. In this step-by-step tutorial, you will see how you can use Selenium to retrieve the verification code provided to the newly subscribed user via SMS and check if it corresponds to the expected one. This will require you an SMS API provider, such as Twilio. Integrating it into your Selenium test to achieve the desired goal only takes a few lines of code.
Prerequisites
Before getting started, you need:
- A Twilio account: If you do not have one yet, sign up for a free here. Follow
this procedure and set up your new
account for SMS in Java. Then, visit the Twilio console page and log in. On this
page, you will see your
Account SID
andAuth Token
values. Store these in a safe place, you will need them later. - A Twilio phone number: you can get a free Canada or USA Twilio phone number by following this procedure. Specifically, click “Get a trial phone number” and then “Choose this Number” in your Twilio console to retrieve it. Otherwise, buy a phone number as described here. This phone number will be used to receive SMS messages.
Now, you need to add the Twilio Java SDK to your project’s dependencies.
If you are a Maven user, place the following lines in your pom.xml
file:
<dependency>
<groupId>com.twilio.sdk</groupId>
<artifactId>twilio</artifactId>
<version>8.9.0</version>
</dependency>
If you are a Gradle user, add the next line in the dependencies block of your build.gradle
file:
implementation group: "com.twilio.sdk", name: "twilio", version: "8.9.0"
You can learn more about how to integrate the Twilio Java SDK in Maven and Gradle here.
Otherwise, you can manually download a pre-built .jar
Twilio Java SDK file from
here and load it as an external library in your project. Follow
this page to learn how to
add a .jar
module dependency in IntelliJ IDEA.
You are now ready to start using Twilio in your Selenium WebDriver Java script.
1. Connecting to the Registration Page
First, use Selenium to connect to the registration page of the website or web app you want to test the SMS workflow. You can achieve this as follows:
import org.openqa.selenium.*;
import org.openqa.selenium.chrome.ChromeDriver;
public class Main {
public static void main(String[] args) {
// setting the system property for the Chrome Driver
System.setProperty("webdriver.chrome.driver", "<the_path_to_your_chrome_driver>");
// initializing the Selenium WebDriver ChromeDriver class
WebDriver driver = new ChromeDriver();
// connecting to the registration page
driver.get("http://localhost/registration-page");
}
}
This is what http://localhost/registration-page
looks like:
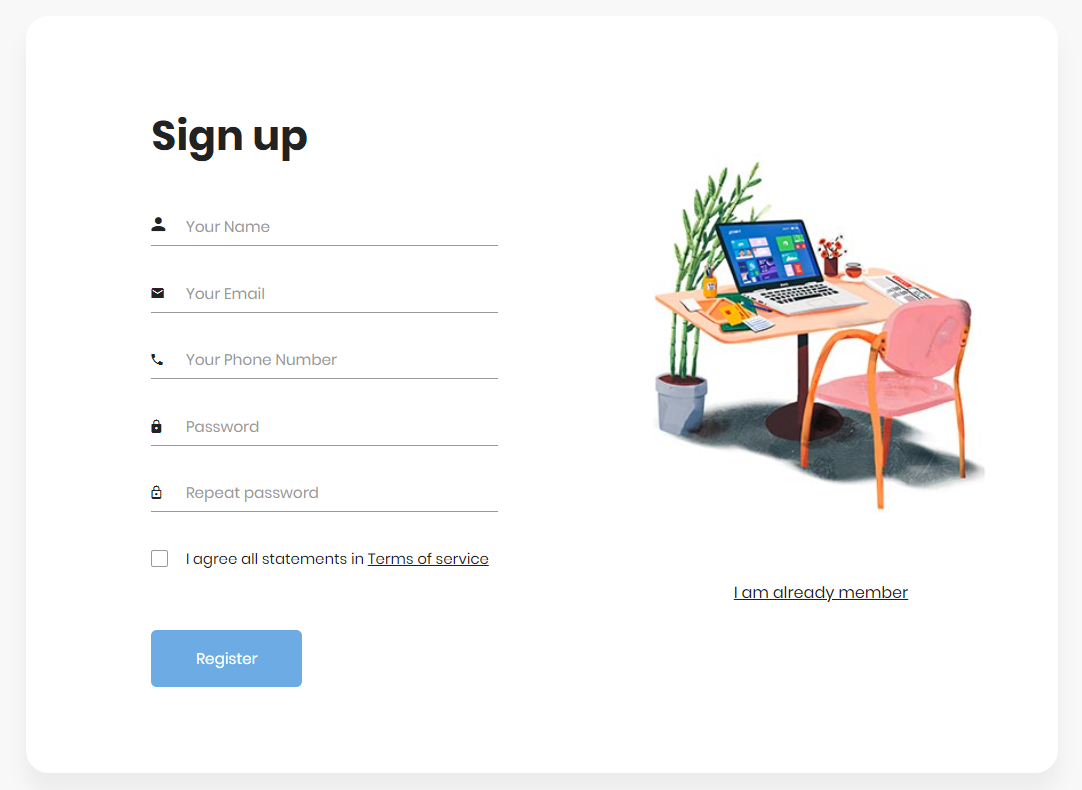
As you can see, it is a simple registration form based on a
free Bootstrap 5 template that requires the user to insert their
phone number. When testing an existing website, replace http://localhost/registration-page
with the actual
registration page URL.
2. Filling out the Registration form
Now, fill out the registration form and submit it with Selenium to trigger the SMS workflow.
This is what the HTML of the sample registration form seen before looks like:
|
|
Therefore, the Selenium script to fill out the form and submit it should implement the following logic:
|
|
This logic works with the aforementioned registration form, but you should adapt it based on the layout of your target
registration page. Also, make sure to replace +1 555 2368
with the Twilio phone retrieved earlier. Otherwise, you will
not be able to use the Twilio APIs to retrieve SMS messages from it.
3. Retrieving SMS messages using Twilio
You can programmatically retrieve the last SMS messages received to your Twilio phone number with the following logic:
|
|
Make sure to replace <YOUR_TWILIO_ACCOUNT_SID>
, <YOUR_TWILIO_AUTH_TOKEN>
, and <YOUR_TWILIO_PHONE_NUMBER>
with the
Account SID, Auth Token, and phone number retrieved earlier from your Twilio Console page. Then, replace
EXPECTED_PHONE_NUMBER
with the actual phone number you expect to receive the confirmation SMS from.
Considering that SMS can take up to minutes to arrive, you should implement
polling logic. Specifically, the logic above tries to
recover the desired SMS several times in a while
loop, waiting 10 seconds between each attempt. This should be enough
to ensure that the SMS you are waiting for will be received.
In detail, the SMS message retrieval logic is based on the
Message class.
This Twilio static class allows you to send or receive SMS messages with your Twilio phone number. In the snippet above,
Message
was used to retrieve the last 5 SMS messages received in the past 10 minutes. Note that looking only for the
last SMS received should not be a good approach, especially if your Twilio phone number is used by other applications.
4. Extracting the confirmation code from the SMS message
This is what the verification SMS looks like:
|
|
You can extract the verification code from the confirmation SMS body string as follows:
|
|
Specifically, the verification code can be extracted from the SMS message getting the text contained between
“FooService:” and “is your phone” with the
substring(int beginIndex, int endIndex)
String
method.
Putting it all together
This is what the full Selenium Java program looks like:
|
|
With the confirmation SMS seen before, this script would return:
|
|
You just retrieved the verification code received via SMS the newly registered user must use to confirm their phone number. Congrats!
If you’re interested in learning how to get automated test coverage for emails, you can find out more in our article on how to test email workflows with Selenium WebDriver.
Conclusion
In this article, you learned what SMS workflows are, why testing them is so important, and what the most common scenarios are when it comes to automated SMS messages. Taking into account how common SMS workflows have become, you need to know how to test them. Here, you learned how to implement a Selenium WebDriver test that triggers an SMS workflow to verify the phone number of a user who has just subscribed to a website. Then, you saw how to use Selenium to retrieve the verification code from the confirmation SMS sent to the phone number of the user and verify if it matches the expected code.
Thanks for reading! We hope that you found this article helpful. Feel free to reach out to us on Twitter with any questions, comments, or suggestions.