Sometimes web pages notify you about the result of an operation or ask you for confirmation through dialog windows. These dialogs will appear on top of whatever is displayed on the current web page and prevent you from performing any other operation until the dialog is dismissed. These dialogs, which are often referred to as “alerts”, cannot be styled and utilize the browser’s default look-and-feel.
In this article, you will learn what a native alert is, what are the most important types of alerts you should be able to deal with, and everything you need to know to interact with them with Selenium WebDriver in Java. But first, let’s delve into Selenium WebDriver.
What is Selenium WebDriver?
Selenium WebDriver is an open-source web framework to perform cross-browser, automated tests. Selenium WebDriver represents a collection of APIs you can use for testing web applications. Because each browser is different, each requires a particular WebDriver implementation. This implementation is called a driver and is responsible for delegating operations to the browser and handling the communication between Selenium and the browser. The list of browsers supported by Selenium WebDriver includes Chrome, Firefox, Safari, Edge, and Internet Explorer.
Selenium WebDriver performs actions on the elements of a web page to verify that the page behaves as expected. This is done by running Selenium WebDriver scripts, which can be written in Java, C#, PHP, Python, and other programming languages.
What is a Native Alert?
Native alerts are also called JavaScript alerts because they are natively supported by the browser through JavaScript. An alert is a small message dialog that appears on top of the browser window. Alerts are generally used to notify users of info or warning, request input, or ask for confirmation. When a native alert fires, the focus is taken away from the current web page and the user is forced to take an action. This means that native alerts prevent users from accessing other parts of the web page until the action required by the alert is performed.
What Types of Native Alerts Exist?
There are a few native alerts, but the following are the most important ones.
1. Message Alerts Simple alerts are fired with the JavaScript
alert()
function. This function tells the browser to
display a dialog box with an optional message and wait until the user dismisses it.
This is what a native message alert created with alert()
looks like:
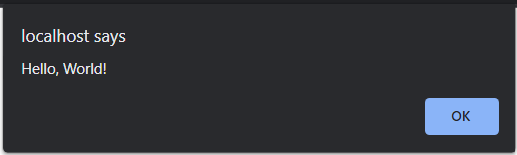
2. Prompt alerts Prompt alerts are fired with the JavaScript
prompt()
function. The function tells the browser to
display a dialog with an optional message prompting the user to input some text and wait until the user either perform
the action or cancels the dialog.
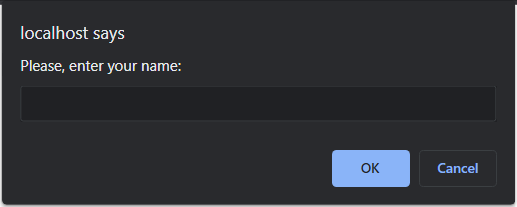
3. Confirmation alerts Confirmation alerts are fired with the JavaScript
confirm()
function. This function tells the browser
to display a dialog with an optional message and wait for the user to confirm or cancel the dialog.
This is what a native confirmation alert created with confirm()
looks like:
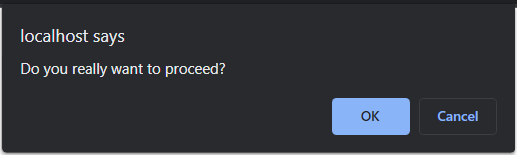
How To Interact With Native Alerts in Selenium WebDriver
Selenium WebDriver equips you with several methods to interact with native alerts in Java. The most important ones are:
-
switchTo()
- Switches from the main window to the alert:1
driver.switchTo().alert();
-
accept()
- Accepts the alert by clicking the ‘OK’ button:1
driver.switchTo().alert().accept();
-
dismiss()
- It dismisses the alert by clicking the ‘Cancel’ button:1
driver.switchTo().alert().dismiss();
-
getText()
- It returns a String containing the alert message:1
String alertMessage = driver.switchTo().alert().getText();
-
sendKeys
- It allows you to send a String to theprompt()
alert input E.g.1
driver.switchTo().alert().setText("Maria Williams");
Now, let’s see how to use them!
- Message Alerts - When dealing with a message alert, you may want to retrieve its message before accepting it. You can achieve this with the Selenium ChromeDriver in Java, as follows:
|
|
- Prompt alerts - When dealing with a prompt alert, you want to provide it with an input value. You can achieve this with the Selenium ChromeDriver in Java, as follows:
|
|
- Confirmation alerts - When dealing with a confirmation alert, you want to accept it or cancel it. You can achieve this with the Selenium Chrome driver in Java, as follows:
|
|
What is the NoAlertPresentException
in Selenium WebDriver?
The previous three above all involve the NoAlertPresentException
. This is a Selenium exception that the driver can
throw on switchTo().alert()
or any other method called on an
Alert
variable. The
NoAlertPresentException
occurs when trying to switch to an alert that is not present at the time of the switch. This
can occur due to unexpected behavior, resulting in test failure.
Selenium might also throw a NoAlertPresentException
when the alert dialog element has not yet been loaded into the
DOM. To avoid this, you can use
WebDriverWait
as
below:
|
|
If the alert is not displayed in 10 seconds after calling the
until()
method, then a
TimeoutException
will be
thrown. The snippet in case of a message alert would become:
|
|
In this case, the try / catch statement on NoAlertPresentException
is no longer required, since no
NoAlertPresentException
will be thrown.
Do other alerts exist? Is it possible to interact with them in Selenium WebDriver?
There are other native alerts in addition to those you can create with the alert()
, prompt()
, and confirm()
JavaScript functions. Let’s learn at how to interact with them in Selenium WebDriver.
-
onbeforeunload alert: The
beforeunload
event is fired by the browser when the window, the HTML document, and its resources are about to be unloaded, but the document is still visible. You can handle this event in JavaScript as below:1 2 3
window.onbeforeunload = function () { // ... };
When this event is handled and fires, each browser will display its own alert. This is what the
onbeforeunload
alert looks like in Chrome:This is just a special confirm dialog, and you can handle it in Selenium WebDriver as explained earlier.
-
HTTP Basic Auth alert: The built-in HTTP standard for restricting access is known as basic authentication. In HTTP basic authentication, a request is authenticated when it contains the following header field:
1
Authorization: Basic <credentials>
<credentials>
is the Base64 encoding of a username and a password joined by a single colon:
.When visiting a web page protected by HTTP Basic Auth with the browser, you are prompted to enter credentials through an alert. Here is what the HTTP Basic Auth alert looks like in Chrome:
This is a special alert, which cannot be traced back to any of the alerts seen before. Fortunately, you can pass username and password directly in the URL with the following syntax:
1
https://username:password@<remaining_part_of_your_url>
So, the line of code to access the web page with the Selenium driver would become:
1
driver.get("https://username:password@<remaining_part_of_your_url>");
When you access a web page protected by HTTP Basic Auth through a URL with valid credentials, you will be logged in directly and no alerts will be shown.
Conclusion
In this article, you learned that Selenium WebDriver is a powerful tool to perform automated tests on web applications, what native alerts and their most important types are, and how to use Selenium WebDriver Java scripts to deal with them. Considering the popularity of native alerts, being able to use Selenium WebDriver to interact with them is critical to building robust tests. Here you learned everything you need to know to interact with them.
Reflect: A testing tool with built-in support for native alerts
Reflect is a no-code testing tool that can test virtually any action you can take on a browser. In addition to testing workflows that require interacting with a native alert, you can also test actions like drag-and-drops, hovers, and file uploads. Creating a test in Reflect is easy: the tool records your actions as you use your site and automatically translates those actions into a repeatable test that you can run any time.
Get automated test coverage for your app today — try it for free.