Looking to create automated tests for your web application? In this guide we’ll walk through how to install and create your first tests using Cypress, an end-to-end testing framework.
Installing Cypress
There are two ways to install Cypress: via the command-line using npm, or by using one of the standalone installers provided for Windows, macOS, and Linux at https://www.cypress.io/. The standalone installer has some limitations, including not being to view the results of your Cypress tests online, so we recommend installing Cypress via npm.
In order to install Cypress via the command line, you’ll first need to make sure you do the following:
-
You must install Node.js on your system. The version for your OS can be downloaded from the Node.js download page. To install Cypress with npm, you need Node.js version 10 or higher.
-
A code editor. We recommend Visual Studio Code, a free code editor created by Microsoft which can be downloaded from the Visual Studio Code homepage.
Method 1 - Installing Cypress via Node Package Manager (npm)
- Open a terminal.
- Create a project directory using the command
mkdir <directory-name>
where “directory-name” is the name you want to give your project. - Navigate to the project directory, by running the command
cd <directory-name>
. Where ‘directory-name’ is the name of the directory created in the previous step. - Run the command
npm init
command. You’ll be prompted to provide a package’s name, author name, description, and keywords. Apackage.json
file is created automatically. - Once you fill in the details, run the following command to install Cypress:
|
|
Cypress is now installed locally in your project directory and is ready to use!
Method 2 - Direct download and Installation of Cypress
If you are unfamiliar with npm but want to use Cypress immediately, download Cypress’s zip file from a Content Delivery Network (CDN). You can use the direct download link to access the updated version of Cypress. This Cypress file, once extracted, can be started quickly without needing to install extra files or plugins.
Now, you have successfully installed Cypress.
Click here to learn how to create a project path on Cypress.
Component vs. end-to-end testing
Cypress supports two types of automated testing: component testing and end-to-end testing. When creating your first project, Cypress will prompt you to choose what type of tests you want to write, so before creating our first test, let’s cover the differences between these two approachest to testing:
What is component testing?
Put simply, component testing is where you test a single component in your UI in isolation. Imagine you’ve developed a
new React component called Foo
. Before using this component in your application, you may want to write some automated
tests that verify that the component is working as expected.
With Cypress’s component testing support, you could write a set of Cypress tests to verify the behavior of the
component. Unlike an end-to-end test, it’s not going to use the component as part of the larger workflow. Instead, the
component tests will test isolated behavior, such as what happens when the Foo
component is clicked.
What is end-to-end testing (E2E)?
End-to-end tests are meant to test complete workflows in an application. They are the closest thing to simulating how an actual user uses your application. Unlike component tests, which test some isolated action, a single end-to-end test may touch many components and exercise lots of different functionality.
Consider an end-to-end test that simulates registering as a new user on your application. Your new Foo
component may
appear on the registration page and is subsequently interacted with as part of the test. But the test may also interact
with many other components and make real network calls.
Now that we’ve covered the differences between E2E and component testing, let’s walk through how you’d create each of these types of tests in Cypress. First, we’ll cover end-to-end tests.
Creating an end-to-end test in Cypress
In order to test Cypress, we’ll first need to set up a sample application. Below we’ll create a minimal ecommerce app that has a product listing page as well as a cart page. From the products page, you can add an item to cart, and then navigate to the carts page to see the added products.
The complete code for this example can be found on GitHub
Step 1: Install node-static-server
In the project directory we created earlier, we will be installing a new package to render static files. This package will be used to run static html files on our server.
|
|
Step 2: Create a server.js
file in the root project directory and add the following contents:
|
|
Step 3: Create 3 new files in the public folder (create this folder if it doesn’t already exist): index.html
,
products.html
, and cart.html
:
index.html
|
|
products.html
|
|
cart.html
|
|
Step 4: Create a new file product-spec.cy.js
inside the cypress/e2e
folder and the content below:
|
|
Step 5: Start your local server
Run the command on your terminal node server.js
to start the server running the app.
Step 6: Run your Cypress tests with the following command:
|
|
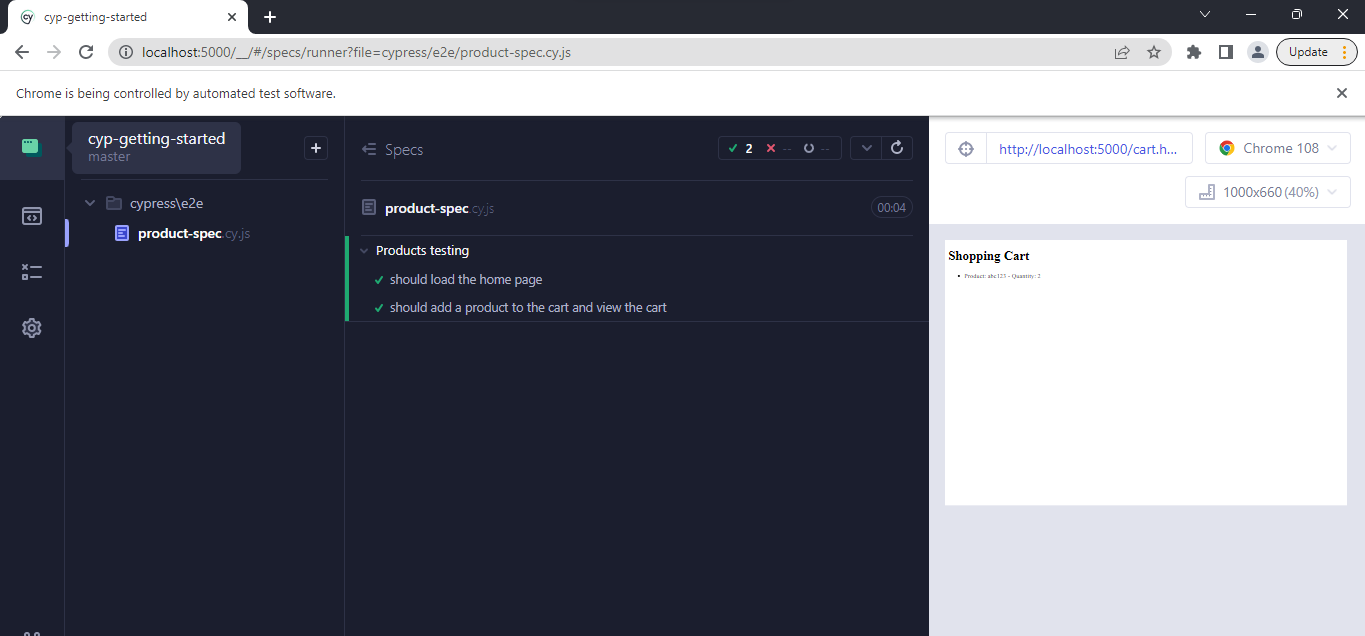
Creating a component test in Cypress
The component test takes each functional part of the software and tests it one at a time. At this time, component testing with Cypress works within the context of a UI framework. React, Vue, Angular, and Svelte are the supported frameworks.
Here is how to create your first component test using the Vue front-end framework. Note that this assumes that you have already created a Vue project.
Step 1: Inside the Vue project, we will create a new component called modal.vue
Step 2: Add the following code to the component file:
|
|
Step 3: Create a Cypress spec file in the /cypress/e2e
folder. The file should have the following content:
|
|
Step 4: Run your Cypress tests
|
|
Frequently Asked Questions
Is Cypress free?
Yes, it’s free and open source. You can run Cypress on your hardware and use an open-source tool called Sorry Cypress to run tests.
What browsers are supported by Cypress?
Cypress supports testing on Chrome and other Chromium-based browsers such as Microsoft Edge, as well as Mozilla Firefox. It also has experimental support for testing in Safari.
How are tests defined in Cypress?
Tests are defined in JavaScript files within the cypress/e2e
directory. Each test case is defined within an it()
block inside the file, and test cases are organized within describe()
blocks which describe the common behavior of the
tests inside it.
Try Reflect: A no-code alternative to Cypress
Reflect is a no-code testing platform that lets you build and run tests across all popular browsers. Reflect doesn’t just make it easy to create tests; it also has robust support for modifying tests and extracting common test steps to be shared across multiple tests. This means that it’s much easier to scale your testing efforts in Reflect compared to other tools.
Looking for a great alternative to Cypress? Try Reflect for free.