Deno is a new runtime environment for JavaScript, TypeScript, and WebAssembly designed to be simple, secure, and efficient. Deno is built on the V8 JavaScript engine and the Rust programming language. Deno aims to be a more modern runtime than Node.js, with better security, performance, and developer productivity support.
Deno Deploy is a platform that makes it easy to deploy your Deno applications. It handles all the details of setting up a production-ready environment, so you can focus on writing your core business logic.
This article will investigate what Deno Deploy is, and how it can help you deploy your Deno applications.
The Deno Runtime
Deno was created by the same person who built Node.js, Ryan Dahl. It’s designed to be more secure and easy to use than Node.js, and it comes with a set of built-in tools that makes it simple to get up and running with Deno.
TypeScript is a superset of JavaScript that adds static type checking and other features that can help improve code quality. Deno includes first-class support for TypeScript, which means that you can write your code in TypeScript and have it automatically compiled to JavaScript when you run it.
One of the critical features of Deno is that it is designed to be secure by default. That means that when you run a Deno program, it will not have access to the file system or network by default. If you want your program to access these resources, you must explicitly grant it permission. This approach helps prevent Deno programs from doing things they’re not supposed to do, like deleting files or accessing sensitive data.
Another essential feature of Deno is that it supports modules. Unlike Node.js, Deno does not use a package manager like NPM to manage dependencies. Instead, it uses the ESM module loader to load modules from URLs. This means you can deploy your Deno applications without installing any additional software. Deno automatically downloads and caches these dependencies when you run the program.
Getting Started With Deno Deploy
Deno Deploy is designed from the ground up for modern JavaScript programming. It strongly integrates cloud infrastructure with V8 Isolates, allowing developers to quickly build optimally distributed web apps. The service runs in 32 regions worldwide and is created by The Deno Company, the same team behind the Deno runtime.
To get started with Deno Deploy, visit https://deno.com/deploy and sign up using your Github account. Once logged in, click on the “New Project” button.
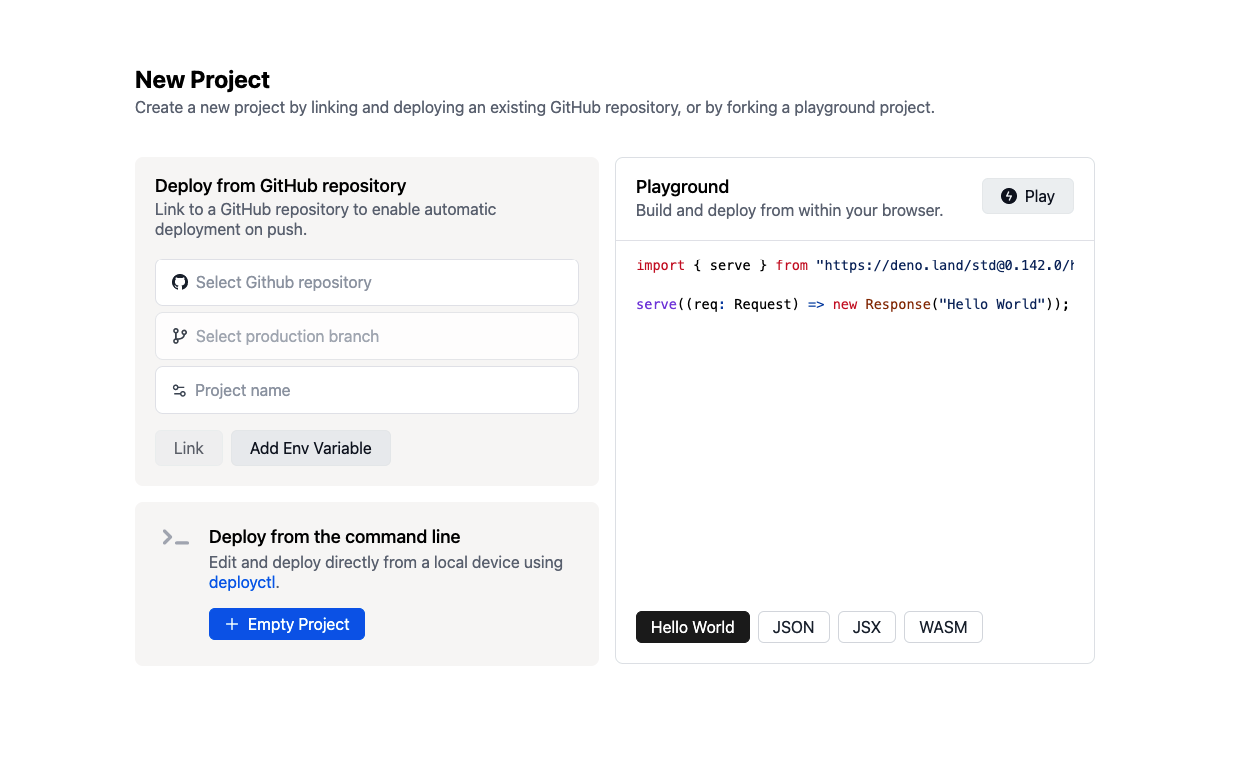
There are three methods that you can follow to deploy your Deno application -
- Using a Github Repo and configuring the project settings.
- Using the
deployctl
command-line tool. - By forking a Playground code snippet, which is an in-browser code editor enabled in your Deno Deploy dashboard.
In this guide we will be using the deployctl
CLI to deploy our app.
Create a new project by clicking on the “Empty Project” button. Once the project is created, you can go to the Settings tab and change the name of the project.
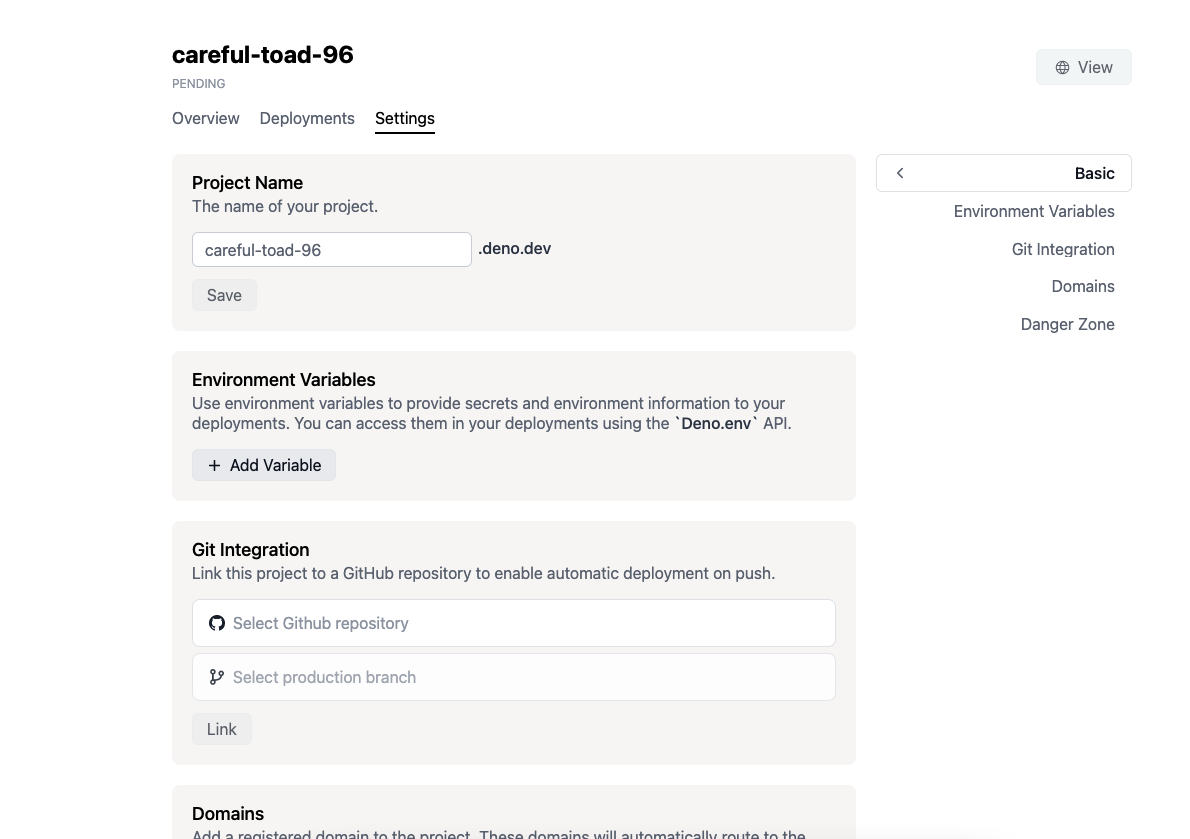
You can also link a Github repo to enable automatic deployments whenever new changes are pushed to the main branch.
Now that the remote project is set up, let’s begin with the code. We will be creating a simple API service that can create and validate UUIDs.
Running Deno Locally
Deno ships with a command-line tool that can be used to run scripts or serve files from a directory. The Deno CLI also provides a way to install packages from third-party sources. You can download the latest Deno release from the official website.
Once you have Deno installed, you can start building web applications and services with it. In this section, you will learn how to deploy a simple Deno application to the web.
Create a new directory for the project and open it up with your preferred IDE.
> mkdir uuid-service
> cd uuid-service
Inside the uuid-service
directory, create an index.ts
file and add the following content.
import * as uuid from "https://deno.land/std@0.119.0/uuid/mod.ts";
console.log(uuid.v1.generate());
Basically here we are using the inbuilt uuid
package for generating the UUID. To run the code, open up your terminal
and type in the following command.
> deno run index.ts
Let’s go ahead and complete the code. We will create a simple handler function that will process the GET
and POST
incoming requests to return and validate the UUID respectively.
import * as uuid from "https://deno.land/std@0.119.0/uuid/mod.ts";
import { serve } from "https://deno.land/std@0.140.0/http/server.ts";
const handler = async (req: Request) => {
const url = new URL(req.url);
if (req.method === "GET") {
const u = uuid.v1.generate();
return Response.json({
uuid: u,
});
}
if (req.method === "POST" && url.pathname === "/validate") {
const body = await req.json().catch(() => null);
if (!body || typeof body?.uuid !== "string") {
return new Response("Bad Request", { status: 400 });
}
const isValid = uuid.v1.validate(body.uuid);
return Response.json({
isValid,
});
}
return new Response("Method Not Allowed", { status: 405 });
};
serve(handler);
To enable access to the network and listen for incoming HTTP requests, you need to explicitly give permission using the
--allow-net
flag while running the Deno program.
> deno run --allow-net index.ts
Test the APIs using the curl commands
> curl --location --request GET 'http://localhost:8000'
> curl --location --request POST 'http://localhost:8000/validate' \
--header 'Content-Type: application/json' \
--data-raw '{
"uuid": "4b392e60-006c-11ed-9550-ddfce82a04be"
}'
Now that your code is working in the local environment, it’s time to deploy it to the cloud platform.
Deploy the code
For deploying the code using the CLI, you’ll require the access tokens from your Deno Deploy profile.
Go to your Deno Deploy dashboard and click on the profile icon → Access Tokens.
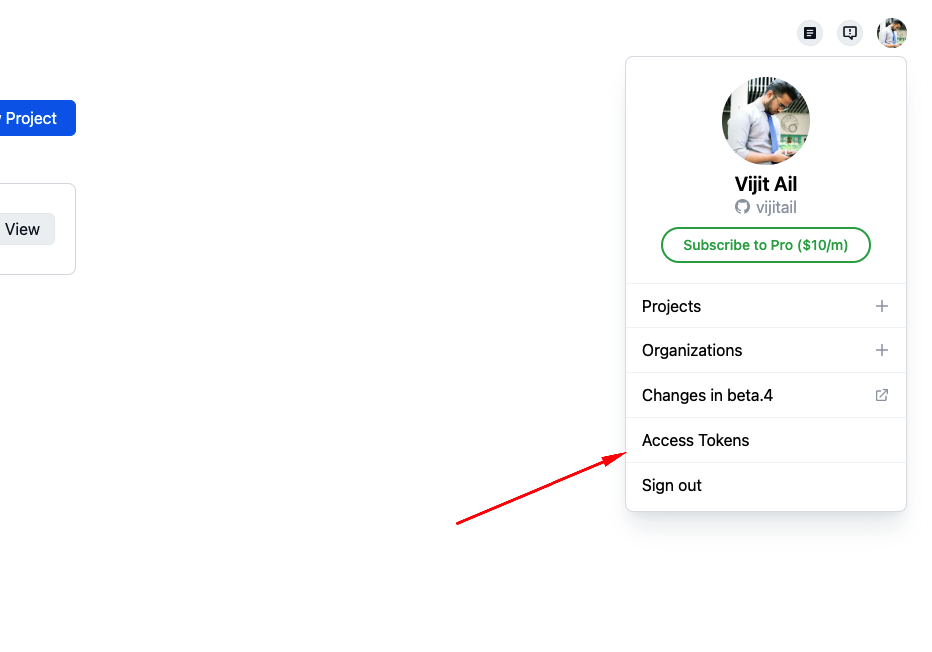
In your Account Settings, click on the “New Access Token” button and generate the access token for the CLI. Make sure you copy the generated token to a secure location as you won’t be able to re-access it in your dashboard.
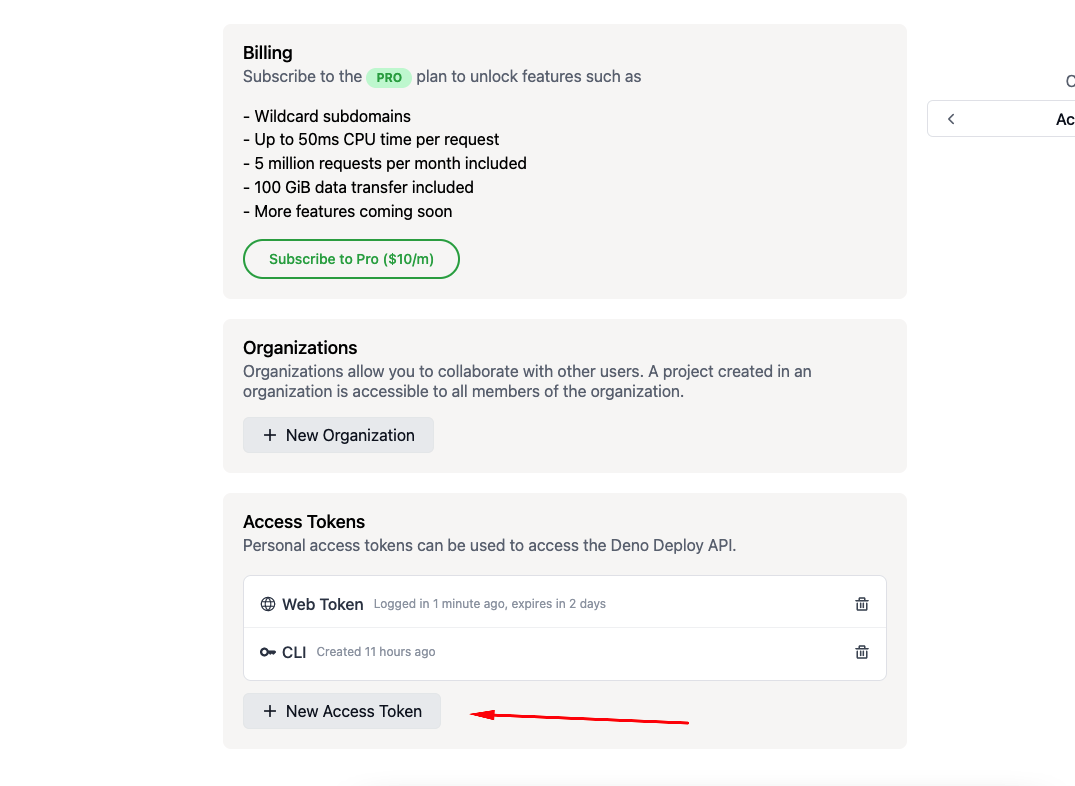
Add the access token in the DENO_DEPLOY_TOKEN
environment variable on your system.
> export DENO_DEPLOY_TOKEN=<YOUR_ACCESS_TOKEN>
And finally you can use the deployctl
CLI to deploy the application using the following command.
> deployctl deploy --project=<PROJECT_NAME> index.ts
Deploy globally with Deno Deploy
With Deno, you can deploy your code anywhere in the world with a single command. As you may have already noticed - there’s no need for complicated infrastructure or platform-specific configurations.
Generally, you may have observed that, when you go to a website, the site’s content may load more quickly or just take longer than you would like. This is because the site might be hosted on servers worldwide, and it depends on where you are located. You will get a much faster response if you are located near the server.
Deno Deploy leverages the edge network to distribute applications worldwide. The edge offers users server locations close to them so they can access the services faster.
The edge network is a system of distributed server locations that work together to automatically identify where your users are located and deliver service to them from the nearest server. You can improve performance and latency by providing service from the edge closest to your user rather than from a central location.
Advantages of Edge Locations
Reduced Latency
Latency is the total time it takes for data to travel from one place to another over a network. The biggest latency issue that arises is because of the physical distance that is present between two points. When the distance between the points is considerable, there are delays and distortion. Latency issues have become almost non-existent since data is sent over edge locations. This also significantly reduces the response time for the end-user.
Availability
Web applications often experience increased traffic during peak periods of activity. To ensure that the web application can handle the increased traffic, it is essential to scale it accordingly. Traditionally, scaling involves adding additional resources, such as servers, to accommodate the high traffic. But with Edge locations managed by Deno Deploy, you don’t have to worry about maintaining servers and scaling them.
Security
Edge locations can also provide security benefits. Storing data in multiple places makes it more difficult for attackers to access or destroy all of it. Edge networks are designed to be resilient against Denial-of-service attacks by malicious users.
Similar Offerings
Deno Deploy is not one-of-a-kind software. As already mentioned, it is a better and more advanced version of Node.js. There are similar offerings in the market like AWS Lambda and Cloudfare Edge Functions.
AWS Lambda
AWS Lambda is a serverless computing service provided by Amazon. Developers can build self-contained functions and applications written in any language the system supports. These functions can perform many tasks, from processing data streams to serving different web pages. They can even be integrated with other AWS functions.
Deno Deploy is a newer option that offers some advantages over AWS Lambda. For one, it’s cheaper – no need to pay for separate hosting or storage costs. Deno Deploy makes deploying multiple versions of your application accessible, so you can easily roll back if there are any issues.
However, AWS Lambda does have some benefits over Deno Deploy. First, it offers more features and integration options. Additionally, AWS Lambda is more widely used, so there’s more support available if you run into problems.
Ultimately, deciding which platform to use depends on your specific needs and preferences. If cost is a significant factor, then Deno Deploy may be the better option. However, AWS Lambda is worth considering if you need more features and integrations.
Cloudflare Workers
Cloudflare is a service that literally works as an accelerator. It can offer website owners and developers a platform to speed up the loading of their websites by caching data from the server, which speeds up the consumer’s experience.
Cloudflare Worker is a JavaScript-based platform that allows developers to host serverless functions. They allow serverless functions to be as close to the end-user as possible and execute whenever they receive an incoming HTTP request. Cloudflare Workers allow the JavaScript code to use all of the browser’s service workers API, which provides an extensive range of functionality.
Unlike Deno, Cloudflare workers do not support TypeScript out-of-the-box.
Conclusion
In this article, we have covered what Deno Deploy is and how it can help you to deploy your Deno applications. We have also looked at some of the benefits of using Deno Deploy, such as the fact that it is easy to use and can automate the deployment process.
Now that you know what Deno Deploy is, it’s time to try it out! This powerful platform can help you quickly and easily deploy your Deno applications. So why wait? Get started today and see how easy it is to use.