Introduction
Testing workflows that include a file upload step can be quite difficult to automate. Popular testing tools like Selenium lack the ability to test file uploads out of the box, but luckily there are third-party tools that make automatically testing file uploads possible.
In this article, we’ll cover how a third-party tool called AutoIT can be used in combination with Selenium to build end-to-end tests that include file upload functionality.
Testing file uploads
Even for something as seemingly straightforward as uploading a file, there are actually quite a few things you could validate as part of an automated test, such as:
Verifying that the file was uploaded correctly. This “happy path” scenario is what most people are looking to test and what oftentimes will provide the most value when it comes to choosing what to automate.
Test file upload privileges based on user permissions. In some cases, file upload behavior is restricted to certain roles within the application. Testing who has access to upload a file can be part of a large strategy for testing role-based access controls or RBAC for short.
Test that only certain file types can be uploaded. Ideally, in these tests, you’re validating that the file’s
extension is checked by your file upload logic and that your system parses the file and rejects files that may have been
renamed with an incorrect extension (e.g., .js
to .jpg
).
Test to be specific to the maximum size file. This type of boundary testing can defend against bugs that threaten the availability of the system since huge files can slow down or even crash a web application.
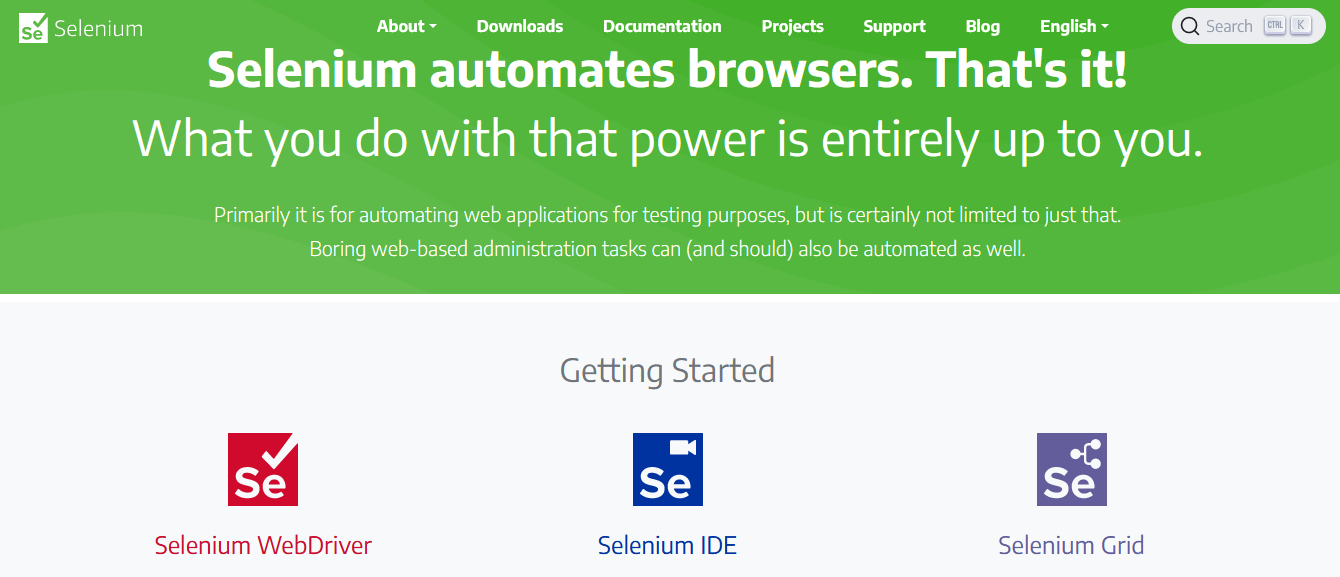
What is Selenium?
Selenium is a popular tool for automating interactions with a browser and is most commonly used for automated testing scenarios.
Despite its popularity and maturity as a software testing tool, Selenium doesn’t have built-in support for file uploads. Since file uploads involve functionality and data that lives outside of the browser, you could argue that file uploads are technically outside of the scope of a tool like Selenium. However, given how common it is to find file upload behavior in web applications, having some way to support testing this behavior can provide a lot of benefits.
By combining Selenium with a tool called AutoIT, we can create automated tests that test end-to-end workflows that include file uploads.
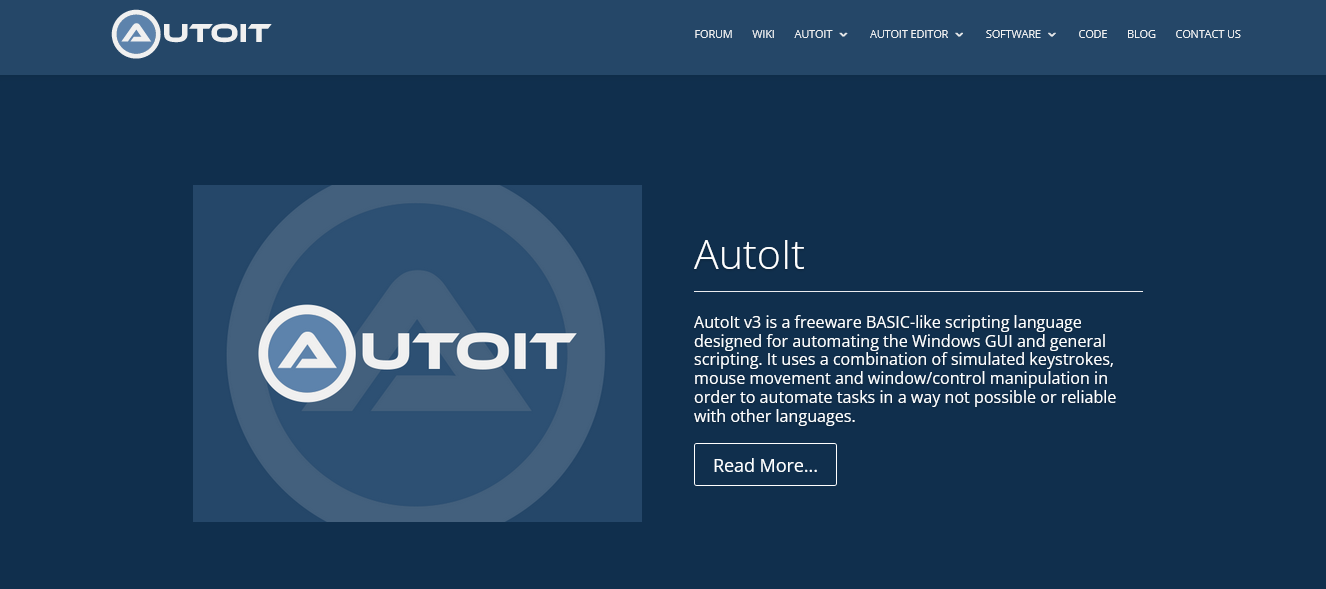
Using AutoIT
AutoIT is “a freeware BASIC-like scripting language” that fills some of the gaps needed to build a full end-to-end test for a file upload. Like Selenium, AutoIT provides tools for triggering mouse clicks and keyboard events. But unlike Selenium, it is built to drive an underlying Windows OS, rather than the web browser process.
This tutorial will use Python, but you can use Java or any other language supported by Selenium.
In AutoIT, we’ll write a simple script that waits for a file upload dialog, inputs a known file, and submits it. Here’s what that looks like:
|
|
In order to make this script triggerable by Selenium, we’ll need to compile it and produce an executable. Using the
Aut2Exe command, we’ll generate an output file called uploadtest.exe
.
For the Selenium test, we’ll want to script the actions leading up to the file upload dialog getting triggered, invoke the AutoIT script we just wrote to complete the file upload process, and optionally add any additional Selenium actions or assertions once the file upload has completed.
Here’s an example Selenium script written in Python that implements these steps:
|
|
Note that in some cases, you can use Selenium’s send_keys()
method to trigger the file upload. This lets you avoid
having to use AutoIT, but you’ll need to test this for yourself on your own application to determine if this approach
works:
|
|
Although AutoIT augments Selenium to provide robust support for testing file uploads, there are still some major limitations:
- AutoIT needs to run on Windows. AutoIT is only compatible with Windows operating systems, so if you’re developing on a Mac or running your tests on Linux, this would not be a viable option.
- It can lead to brittle tests. Since we’re scripting the Windows UI itself, there can be cases where the script fails due to some change or non-deterministic behavior within the Windows environment.
- You’ll still need to host the files yourself. Since AutoIT requires the file to be accessible via a system drive, you’ll need to figure out a way to ensure the file exists at the proper location on the system running the tests. For a solo dev doing local testing, this isn’t too difficult, but if you’re on a team and/or are running tests on a continuous integration service like Jenkins or CircleCI, then this gets significantly more complex.
Conclusion
Testing file uploads in Selenium is possible through the use of third-party tools like AutoIT. Just be aware of the potential pitfalls of using these technologies to test file uploads, especially when it comes to test flakiness and creating tests that work on local machines and CI servers. Despite its challenges, having an automated approach for testing file uploads can provide test coverage for many critical workflows and thus be very important in your overall regression testing strategy.
Reflect: A testing tool with built-in support for testing file uploads
Reflect is a no-code testing tool that can test virtually any action you can take on a browser, including file uploads. Creating a file upload test in Reflect is easy: the tool records your actions as you interact with the application and automatically turns those actions into a repeatable test. For file upload steps, files are saved securely in the cloud so they can be re-used each time the test runs.