In Selenium, a selector, which is also called a locator, is what’s used to identify elements within a web page. Selenium supports two types of selectors: CSS selectors, and XPath selectors. In Java, when an invalid CSS or XPath selector is malformed, Selenium throws an InvalidSelectorException. This exception represents one of the most common Selenium exceptions, and chances are that you’ve already encountered it if you’ve used Selenium for any length of time.
In this article, we’ll cover under what conditions Selenium throws a InvalidSelectorException
, and how you can avoid
it when writing selectors in your Selenium WebDriver tests.
What is an InvalidSelectorException?
Selenium throws an InvalidSelectorException
when an XPath or CSS selector does not conform to the XPath or CSS
specification. In other words, an InvalidSelectorException
occurs when you pass in a selector which can’t be parsed by
Selenium WebDriver’s selector engine.
InvalidSelectorException
is the sole subclass of
NoSuchElementException,
which is another common exception that indicates when targeted element cannot be located. If you’re curious when you
might encounter other instances of NoSuchElementException
, check out our other article on
how to deal with NoSuchElementExceptions in Selenium.
Below is an example Selenium test that contains a findElement
call that throws an InvalidSelectorException
:
|
|
The CSS selector used in this example is attempting to find an element with a value
attribute that has the value
active
, however it’s using curly braces, which is not supported in CSS selectors. This selector should instead use
square brackets (e.g. [value='active']
). When running the test, you’ll see the following exception message in your
console: InvalidSelectorException
:
|
|
When does Selenium throw an InvalidSelectorException?
The two most commons reasons for an InvalidSelectorException
are that the selector is malformed, or it’s using a new
feature not supported in the current version of Selenium WebDriver. We’ll cover examples of both issues below:
Reason #1: Malformed selectors
There are many possible variants of a malformed selector. Below is a trivial example of an XPath selector which cannot be parsed:
|
|
The following exception will be displayed to the console:
|
|
We can produce a similar result for a CSS selector:
|
|
This will return the following error:
|
|
Reason #2: Using a selector feature not supported in WebDriver
The other common reason for hitting an InvalidSelectorException
is that you’re using a feature of CSS or XPath that’s
not supported by the underlying browser engine, and thus not supported by Selenium WebDriver.
This is a more common problem with XPath selectors than CSS selectors. Since modern browsers only support the XPath 1.0
spec, which was released over twenty years ago, there are many XPath features that can be found online but which will
never work in a web browser. The example below uses the contains
function, which is a feature introduced in XPath 2.0
and thus not supported in Selenium:
|
|
This will fail with the following exception message:
|
|
Unlike XPath, browser engines have done a much better job at keeping up with the CSS spec. There have thus far been 4
versions of the CSS spec. While browser engines have 100% coverage of CSS v3, as of this writing browser engines have
roughly 65% coverage for the version 4 spec. This means that if you’re attempting to use bleeding-edge CSS syntax, you
may hit an InvalidSelectorException
.
Below is an example that references the :blank
pseudo-class, which is not currently supported in Selenium WebDriver:
|
|
Using this selector will result in the following error:
|
|
Other potential causes
While the two issues described above are the most common reasons for this exception, there could be other potential causes, including:
- When using the deprecated
By.tagName
method, an
InvalidSelectorException
is thrown if you pass an empty string. - When using the
By.className
method, an
InvalidSelectorException
is thrown if you pass in more than one classname (e.g."foo bar"
). Compound class names are supported when usingBy.cssSelector
.
Preventing InvalidSelectorExceptions
Since Selenium relies on the browser’s capabilities to find an HTML element with an XPath or CSS locator strategy, the best way to avoid invalid selectors is to first test your selectors in the browser prior to putting them in your Selenium tests. Using Chrome Developer Tools, you can test any XPath or CSS selector on a web page directly. Let’s learn how.
Validating XPath Selectors in the browser
You can test if an XPath selector is valid by passing it in the $x
function that comes built-into Chrome Developer
Tools. Open Chrome Dev Tools, switch to the Console tab, and paste the following code below which will return the
<html>
tag from the current webpage:
|
|
This is a special function available in Chrome Dev Tools. Note that this behavior is defined in the W3C WebDriver spec here.
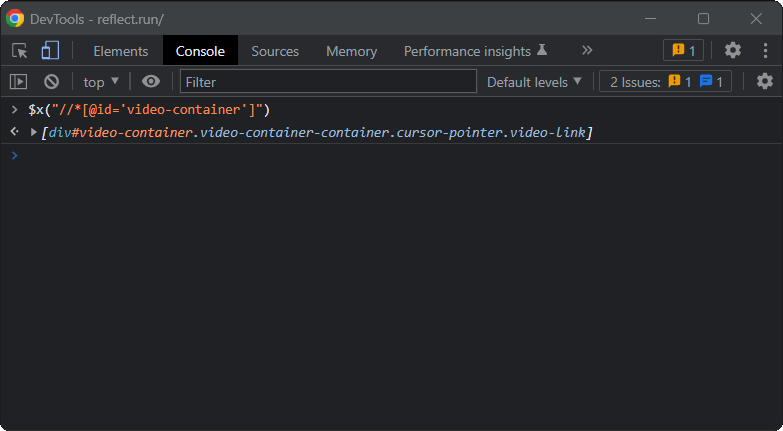
Looking for XPath locator on the reflect.run homepage
If the XPath selector is valid, the function will return an array of HTMLElements selected by that selector. Otherwise, it will return the following error:
|
|
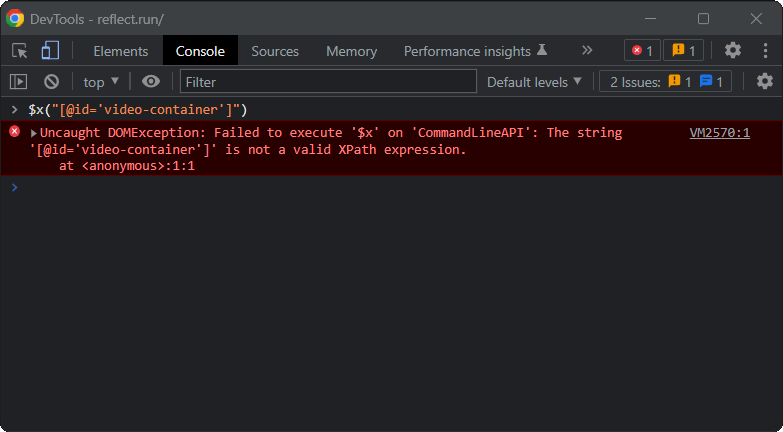
Validating CSS Selectors in the browser
You can verify whether a CSS selector is valid by calling the following line of code in the Console tab within Chrome Developer Tools:
|
|
For example:
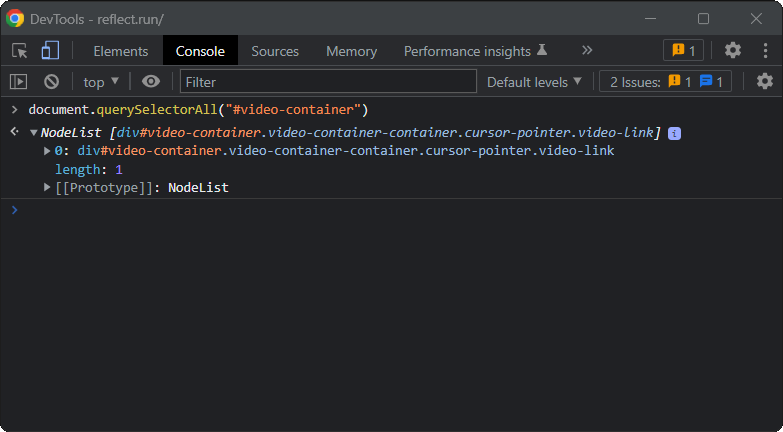
Looking for CSS selector on the reflect.run homepage
If the CSS selector is valid, the command will return an array of HTML elements identified by that selector. Otherwise, it will produce the following error:
|
|
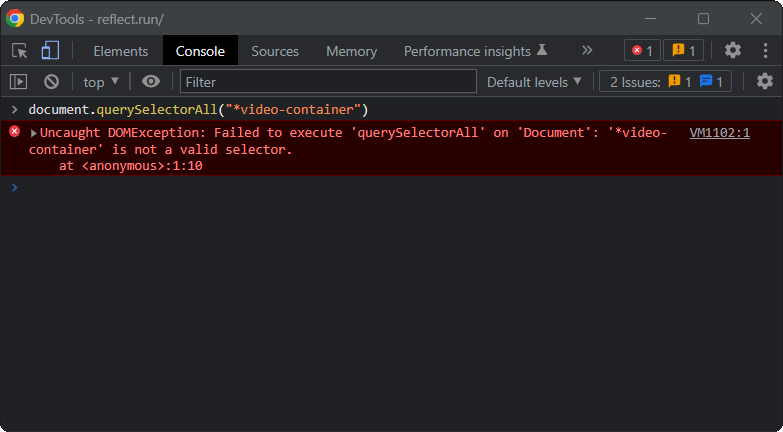
Similarly, you can use the following shorthand function:
|
|
This is a function exposed by Chrome Dev Tools by default. Note that this behavior is defined in the W3C WebDriver spec here.
On valid CSS selectors, it returns a list of HTMLElements
. Otherwise, it raises the following error:
|
|
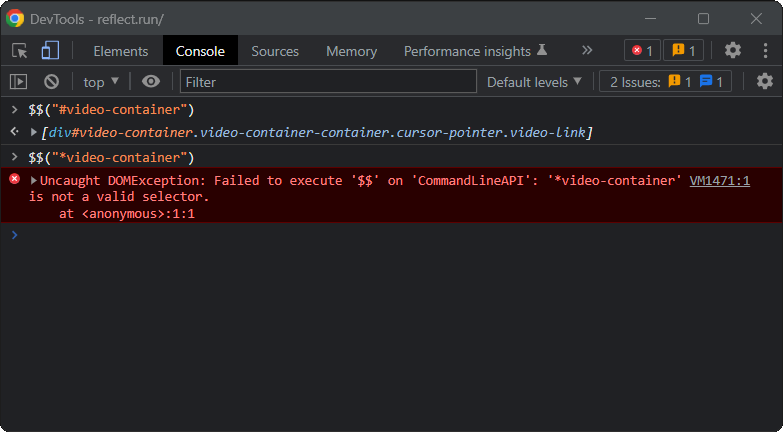
Frequently Asked Questions
What version of XPath is used by Selenium WebDriver?
Selenium WebDriver uses the browser’s native XPath capabilities wherever possible. Even though never versions of XPath are available, modern browsers only support the XPath 1.0 standard.
XPath 1.0 was released in 1999. This is the version that all modern browsers support. XPath 2.0 in 2010, while the latest version of XPath is 3.1 was released in 2017.
If you’re testing against legacy browsers (i.e. Internet Explorer 8 and below), Selenium WebDriver provides its own implementation of XPath, which is also based on the 1.0 standard.
In other words, if you are using any features added to XPath in the last 20 years, you are going to hit an
InvalidSelectorException
.
What version of the CSS Selectors spec is used by Selenium WebDriver?
Selenium WebDriver uses the browser’s native CSS selector capabilities. Level 4 was released in May 2022 and is the latest version of the CSS spec.
Not all browsers support Level 4 CSS selectors features, and some of them support Level 4 only partially. This means
that using a Level 4 CSS selectors feature may or may not result in a InvalidSelectorException
.
I recommend using this online tool to check how well your browser supports the CSS selectors from Level 1 up to Level 4. For example, Chrome 106 supports 100% of Level 1, 100% of Level 2, 100% of Level 3, and 65% of Level 4.
Auto-generate selectors using Reflect
To interact with an element on a web page with Selenium, you must first select it. As seen above, defining the right selector is not always easy. Plus, that requires you to be able to understand the source code of a web page. What if you could select a web element with a simple click? That would take away all the hassle, avoid exception handling, and allow even non-technical users to create automated tests.
Reflect is a no-code testing tool that allows you to create tests for your web applications through an intuitive, simple-to-use, point-and-click interface.
Assume you want to verify that a particular element in your page contains the expected text. With Selenium, you would have to manually inspect the source code of the page, define a valid selector, extract the text from it, and finally compare it with the target test value. That is a cumbersome task that only experienced developers can accomplish. With Reflect, you only have to select the text validation option and then click on the desired element directly in the browser.
Behind the scenes, Reflect automatically generates and uses the right selectors. This eliminates the tedium of writing selectors for each test step and keeping them updated over time. Avoid struggling with errors in your tests, try Reflect for free!
Conclusion
In this article, you learned what a InvalidSelectorException
is, why and when Selenium throws it, and you can avoid
it. Considering how frequent the InvalidSelectorException
is, understanding how it happens and being able to debug it
properly is essential to building robust Selenium WebDriver tests. Here, you also had the opportunity to learn more
about the version of XPath and CSS selector specification supported by Selenium WebDriver. Finally, you realized that
handling exceptions during testing can be difficult and can you avoid it by embracing a powerful, complete, no-code
testing tool like Reflect.