There have been lots of Cypress features over the years. They include Cypress Test Runner; this allows developers to see all test files and which browsers are available for running tests. Another is the Cypress code coverage feature, which lets you measure code coverage to know which parts of your code are tested.
In this article, we will look at one of Cypress’ experimental features, Cypress Studio. We will learn about it and how to create and modify tests with Cypress Studio. We will also look at some limitations of Cypress studio and why Cypress removed it in its version 10 release.
What is Cypress Studio
Cypress Studio is an experimental Cypress feature that allows you to develop automated tests with minimal coding. It does this by recording your interactions with the application under test and turning those actions into an equivalent Cypress test. Most of the actions required to interact with your application, such as clicking buttons, typing strings into an input field, checking radio buttons, and picking options from a drop-down menu, are supported by Cypress Studio.
One of the key features of Cypress Studio is its Selector Playground which aids in locating the appropriate selector for each action in the test and lets you avoid writing selectors manually or generating selectors using the Element panel in Chrome Developer Tools.
Installing Cypress
This section will cover how to install Cypress and get Cypress Studio working so you can begin testing with it. Open the application you want to test in your favorite code editor.
Next, open a terminal, navigate to the project and type in the command below to install Cypress:
|
|
The above command saves Cypress as a dev-dependency and will add a bunch of Cypress-related folders to your app.
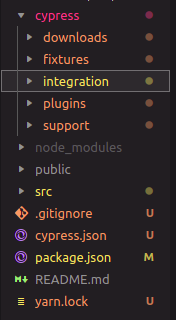
Inside your project’s cypress/integration/
folder, create a new test file and give it a name that ends in .spec.js
.
Now let’s start creating our first test. We’ll start by giving Cypress the URL of the application we want to test:
|
|
To run the test, execute the following command in your terminal:
|
|
Alternatively, you can set this command as the value for the “test” script in your package.json and run it via the
command npm test
.
Getting started with Cypress Studio
Please note that to use Cypress Studio, you must have a version of Cypress installed that is between version 6.3.0 and version 9.7.0. This is because Cypress Studio was released in Cypress version 6.3.0 and was removed in Cypress version 10. If you used the command above to install Cypress, you’ll have version 9.7.0 installed and should see the following in your package.json file.
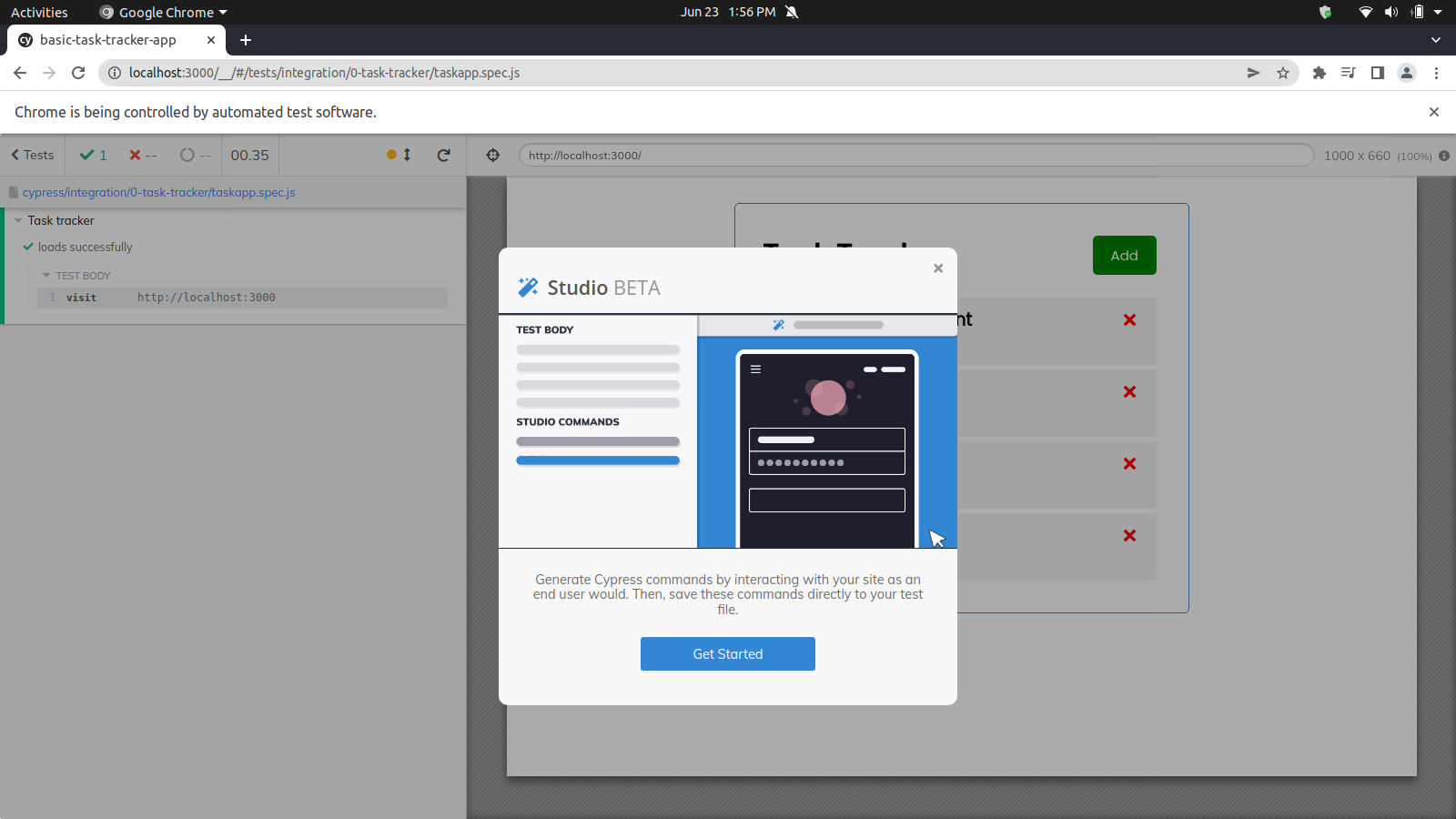
Since Cypress Studio is an experimental feature, you’ll need to explicitly enable it in Cypress’s configuration to try it out. Navigate to your cypress.json file and add the following line:
|
|
Next, you will notice a Cypress Studio icon in your test runner. This icon only appears when you hover on the test name. This is all the configuration needed to get Cypress Studio working.
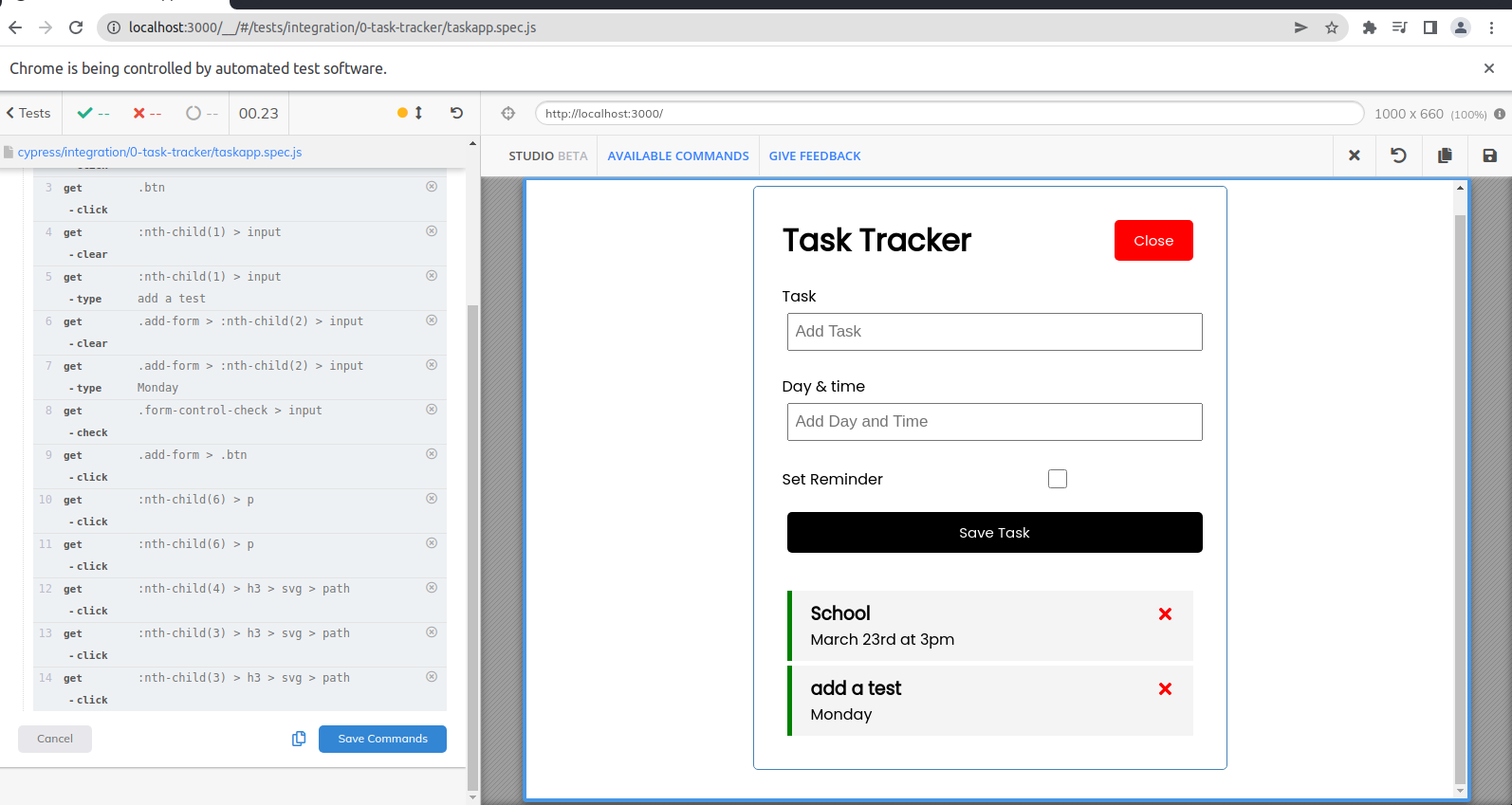
How to create a simple test with Cypress Studio
As soon as you click on the icon, the test runner will go into “recording mode,” allowing you to begin using your app. Actions like clicks, keystrokes, and unchecking or ticking checkboxes will all be recorded. If you perform an action that you don’t want to be included, you can undo that action.
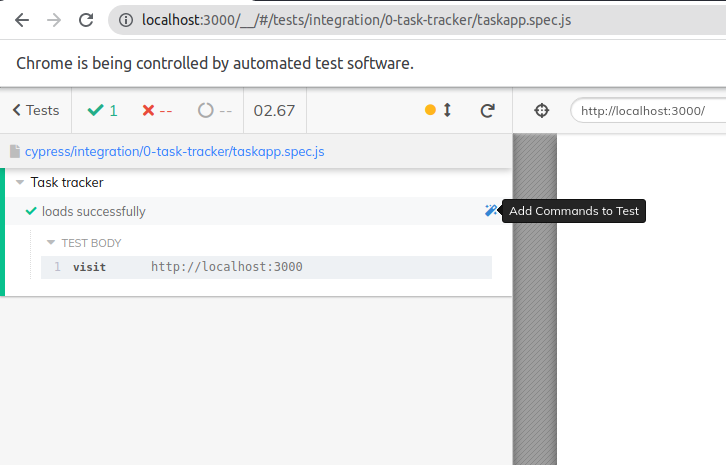
Once you click on get started, you interact with your application as you usually would. As you interact with the application, some tests will get generated on Studio’s Command view, as seen in the image below:
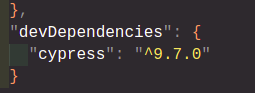
You have the option of either copying or saving these commands. If you save the test commands, you automatically have a test that will always pass. This is very helpful if you only need to quickly and easily verify a functionality.
Navigate to your test file, which ends in .spec.js,
and you will see a Cypress test that contains your auto-generated
actions. Here is an example test that I created using Cypress Studio:
|
|
By default, Cypress Studio does not record any assertions. This means that for your generated code to actually test something, you will need to add some assertions yourself. One way to do this is by adding the assertions directly in the generated code. Here’s an example:
|
|
The other way to add an assertion is via the Cypress Studio GUI. To add an assertion within Studio, you can right-click on the element you wish to assert and then select from a list of assertions that Cypress Studio supports.
How to modify a Cypress Studio test
We have seen that Cypress Studio can make the test creation process faster and simpler. But what about modifying an existing test? Unfortunately, Cypress Studio does not support editing an existing test through the record-and-play workflow. This means that to edit an existing Cypress Studio test, you must do it within the generated code itself.
Let’s say we wanted to modify the test above to include some additional assertions, as well as include actions which are not supported by Cypress Studio. We would need to do that directly in the code. Here’s an updated example with those additional actions and assertions added:
|
|
Limitations of Cypress Studio
Cypress Studio is great for beginners who are taking their first steps into test automation, or for developers who are looking to spend less time building out tests. However, you should be aware of the main limitations of this tool, which we’ll cover below:
Some actions cannot be recorded
Cypress Studio can only detect a subset of the commands that is supported by vanilla Cypress. The commands that Cypress Studio can support are listed below::
.click()
.type()
.check()
.uncheck()
.select()
This means that actions like hovering and drag-and-drop cannot be recorded automatically, and must be added by hand to the generated code.
API stubbing and mocking are not well supported
With more elaborate tests, you might need to stub some API requests and intercept network connections. For Cypress Studio to use these mocked APIs, they must be first defined in a before or beforeEach block prior to recording the test. It would be handy to be able to mock API requests on the fly while recording or capture network traffic while recording to make it easier to build out mocks, but unfortunately, this is not supported.
Cypress Studio and Cypress V10
The Cypress team has announced that they will be discontinuing support for Cypress Studio. This means that starting in Cypress 10, Cypress Studio is no longer included as an experimental feature. The Cypress team provided some additional information in a Github Issue for their decision to remove record-and-playback as a feature of their product. A few reasons for the removal was that the cost of refactoring Cypress Studio to ship with the latest version of Cypress is too high. Another is that it would have prevented the Cypress team from meeting the community expectations for the latest version of Cypress.
Try Reflect: A no-code alternative to Cypress Studio
Reflect is a no-code testing platform that lets you build and run tests across all popular browsers. Reflect doesn’t just make it easy to record tests; it also has robust support for modifying tests and extracting common test steps to be shared across multiple tests. This means that it’s much easier to scale your testing efforts in Reflect compared to other tools.
Looking for a great alternative to Cypress Studio? Try Reflect for free.